From cppreference.com. Typically a declaration of a loop counter variable with initializer. Compilers are permitted to remove such loops. While in C, the scope of the init-statement and the scope of statement are one and the same, in C the scope.
The most common form of loop in C++ is the for loop. The for loop is preferred over the more basic while loop because it’s generally easier to read (there’s really no other advantage).
The for loop has the following format:
The for loop is equivalent to the following while loop:
Execution of the for loop begins with the initialization clause, which got its name because it’s normally where counting variables are initialized. The initialization clause is executed only once, when the for loop is first encountered.
Execution continues with the conditional clause. This clause works just like the while loop: As long as the conditional clause is true, the for loop continues to execute.
After the code in the body of the loop finishes executing, control passes to the increment clause before returning to check the conditional clause — thereby repeating the process. The increment clause normally houses the autoincrement or autodecrement statements used to update the counting variables.
The for loop is best understood by example. The following ForDemo1 program is nothing more than the WhileDemo converted to use the for loop construct:
The program reads a value from the keyboard into the variable nloopCount. The for starts out comparing nloopCount to 0. Control passes into the for loop if nloopCount is greater than 0. Once inside the for loop, the program decrements nloopCount and displays the result. That done, the program returns to the for loop control.
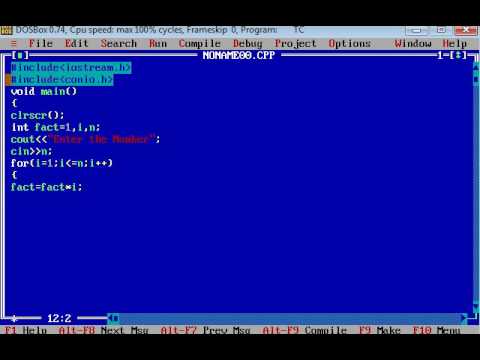
Control skips to the next line after the for loop as soon as nloopCount has been decremented to 0.
All three sections of a for loop may be empty. An empty initialization or increment section does nothing. An empty comparison section is treated like a comparison that returns true.
This for loop has two small problems. First, it’s destructive, in the sense that it changes the value of nloopCount, “destroying” the original value. Second, this for loop counts backward from large values down to smaller values. These two problems are addressed by adding a dedicated counting variable to the for loop. Here’s what it looks like:
This modified version of ForDemo loops the same as it did before. Instead of modifying the value of nLoopCount, however, this ForDemo2 version uses a new counter variable.
This for loop declares a counter variable i and initializes it to 0. It then compares this counter variable to nLoopCount. If i is less than nLoopCount, control passes to the output statement within the body of the for loop. Once the body has completed executing, control passes to the increment clause where i is incremented and compared to nLoopCount again, and so it goes.
The following shows example output from the program:
When declared within the initialization portion of the for loop, the index variable is known only within the for loop itself. Nerdy C++ programmers say that the scope of the variable is limited to the for loop. In the ForDemo2 example just given, the variable i is not accessible from the return statement because that statement is not within the loop.
- C++ Basics
- C++ Object Oriented
- C++ Advanced
- C++ Useful Resources
- Selected Reading
There may be a situation, when you need to execute a block of code several number of times. In general, statements are executed sequentially: The first statement in a function is executed first, followed by the second, and so on.
Programming languages provide various control structures that allow for more complicated execution paths.
A loop statement allows us to execute a statement or group of statements multiple times and following is the general from of a loop statement in most of the programming languages −
C++ programming language provides the following type of loops to handle looping requirements.
Sr.No | Loop Type & Description |
---|---|
1 | while loop Repeats a statement or group of statements while a given condition is true. It tests the condition before executing the loop body. |
2 | for loop Execute a sequence of statements multiple times and abbreviates the code that manages the loop variable. |
3 | do...while loop Like a ‘while’ statement, except that it tests the condition at the end of the loop body. |
4 | nested loops You can use one or more loop inside any another ‘while’, ‘for’ or ‘do..while’ loop. |
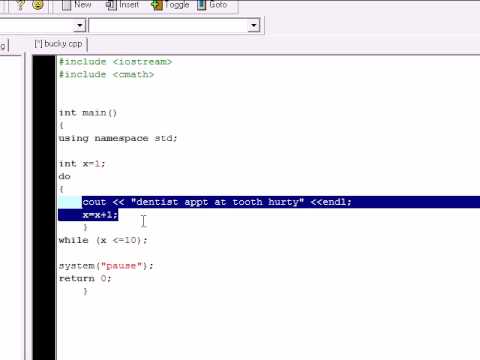
Loop Control Statements
C# Array
Loop control statements change execution from its normal sequence. When execution leaves a scope, all automatic objects that were created in that scope are destroyed.
C++ supports the following control statements.
Sr.No | Control Statement & Description |
---|---|
1 | break statement Terminates the loop or switch statement and transfers execution to the statement immediately following the loop or switch. |
2 | continue statement Causes the loop to skip the remainder of its body and immediately retest its condition prior to reiterating. |
3 | goto statement Transfers control to the labeled statement. Though it is not advised to use goto statement in your program. |
The Infinite Loop
A loop becomes infinite loop if a condition never becomes false. The for loop is traditionally used for this purpose. Since none of the three expressions that form the ‘for’ loop are required, you can make an endless loop by leaving the conditional expression empty.
For Loop Counter
When the conditional expression is absent, it is assumed to be true. You may have an initialization and increment expression, but C++ programmers more commonly use the ‘for (;;)’ construct to signify an infinite loop.
Dev C For Loop Counter For Sale
NOTE − You can terminate an infinite loop by pressing Ctrl + C keys.